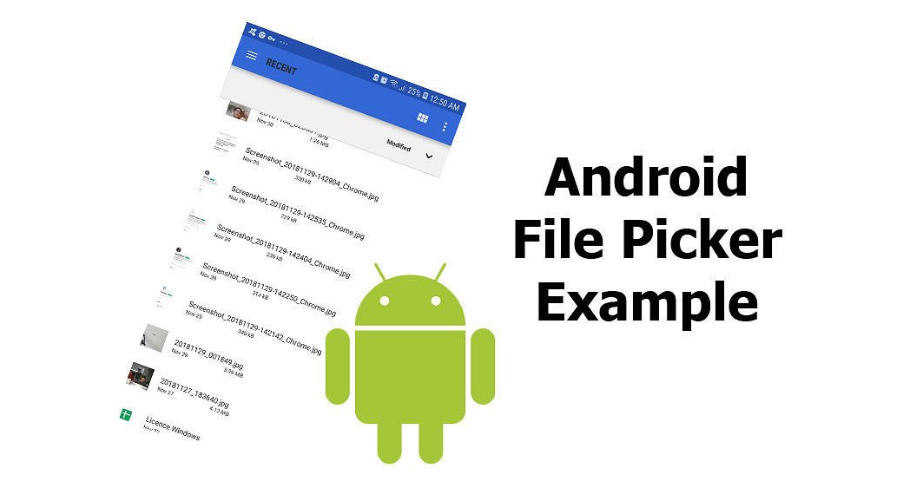
Android File Picker, Do you want to show document picker to your Android. Software to allow customers choosing report in their neighborhood storage? This educational indicates you the way to reveal. A simple document picker and get the File object of decided on file.
Layout
First, create the XML layout. To preserve this tutorial simple, there may be best a Button. So that it will trigger to expose the file picker whilst it’s clicked and a TextView for showing the direction of decided on file.
choose_file.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/fragment_item_edit_content"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="20dp" >
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<Button
android:id="@+id/btn_choose_file"
android:layout_width="@dimen/btn_width"
android:layout_height="@dimen/btn_height"
android:layout_marginLeft="@dimen/btn_spacing"
android:background="@drawable/shape_button"
android:text="@string/item_choose_file"
android:textColor="@color/white" />
<TextView
android:id="@+id/tv_file_path"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
Handle File Picker
In the hobby or fragment. We want to handle while the button is clicked. And while a record has been chosen through person. I use fragment in this situation. We need to add setOnClickListner internal which a brand new ACTION_GET_CONTENT Intent is created. Then name startActivityForResult with the Intent as the first parameter and a request code as the second paramter. The request code desires to be particular to distinguish it from other hobby outcomes. In order to get the result after consumer deciding on report, we need to address it via overriding onActivityResult.
FilePickerFragment.java
public class FilePickerFragment extends Fragment {
public static final int PICKFILE_RESULT_CODE = 1;
private Button btnChooseFile;
private TextView tvItemPath;
private Uri fileUri;
private String filePath;
@Override
public View onCreateView(final LayoutInflater inflater, final ViewGroup container,
final Bundle savedInstanceState) {
rootView = inflater.inflate(R.layout.choose_file, container, false);
btnChooseFile = (Button) rootView.findViewById(R.id.btn_choose_file);
tvItemPath = (TextView) rootView.findViewById(R.id.tv_file_path);
btnChooseFile.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent chooseFile = new Intent(Intent.ACTION_GET_CONTENT);
chooseFile.setType("*/*");
chooseFile = Intent.createChooser(chooseFile, "Choose a file");
startActivityForResult(chooseFile, PICKFILE_RESULT_CODE);
}
});
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
switch (requestCode) {
case PICKFILE_RESULT_CODE:
if (resultCode == -1) {
fileUri = data.getData();
filePath = fileUri.getPath();
tvItemPath.setText(filePath);
}
break;
}
}
}
Get the File Object
With the above code, we are able to get the course to the record, however no longer the file item. In order to get the report object. We want the person to supply permission for the software to study external garage. For Android 5.X and under, we will genuinely upload the permission in AndroidManifest.Xml.
AndroidManifest.xml
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Since Android 6.0, each permissions is requested at the same time. As the application is walking with the aid of showing a popup. That’s what we have to do. To make it smooth, we will use EasyPermission.
First, add the library on your assignment dependency.
app/build.gradle
compile 'pub.devrel:easypermissions:0.2.0'
FilePickerFragment.java
public class FilePickerFragment extends Fragment implements EasyPermissions.PermissionCallbacks {
@Override
public void onCreate(final Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (!EasyPermissions.hasPermissions(getActivity(), Manifest.permission.READ_EXTERNAL_STORAGE)) {
EasyPermissions.requestPermissions(this, getString(R.string.permission_read_external_storage), EXTERNAL_STORAGE_PERMISSION_REQUEST_CODE, Manifest.permission.READ_EXTERNAL_STORAGE);
}
}
@Override
public void onPermissionsGranted(int requestCode, List perms) {
// Add your logic here
}
@Override
public void onPermissionsDenied(int requestCode, List perms) {
// Add your logic here
}
}
Having get the file’s Uri from the previous step and permission. To get entry to external storage, it’s very simple to get the File item.
File file = new File(fileUri.getPath());
That’s all approximately this tutorial of how to show a simple report picker in Android and get the File item.