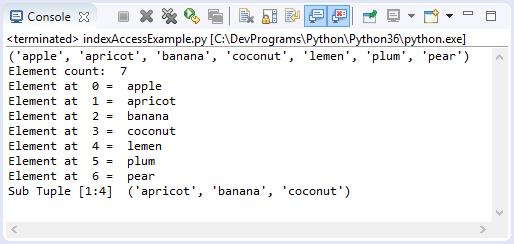
Python – Tuples, A tuple is a chain of immutable Python objects. Tuples are sequences, similar to lists. The differences among tuples and lists are, the tuples can’t be changed unlike lists and tuples use parentheses, while lists use square brackets.
Creating a tuple is as easy as placing unique comma-separated values. Optionally you could put these comma-separated values among parentheses also. For example −
tup1 = ('physics', 'chemistry', 1997, 2000);
tup2 = (1, 2, 3, 4, 5 );
tup3 = "a", "b", "c", "d";
The empty tuple is written as parentheses containing nothing −
tup1 = ();
To write a tuple containing a unmarried cost you have to include a comma, even though there may be handiest one fee −
tup1 = (50,);
Like string indices, tuple indices begin at zero, and that they may be sliced, concatenated, and so forth.
Python – Tuples, Accessing Values in Tuples
To access values in tuple, use the rectangular brackets for reducing along side the index or indices to obtain fee to be had at that index. For example −
#!/usr/bin/python
tup1 = ('physics', 'chemistry', 1997, 2000);
tup2 = (1, 2, 3, 4, 5, 6, 7 );
print "tup1[0]: ", tup1[0];
print "tup2[1:5]: ", tup2[1:5];
When the above code is finished, it produces the following result −
tup1[0]: physics
tup2[1:5]: [2, 3, 4, 5]
Updating Tuples
Tuples are immutable which means that you can not update or alternate the values of tuple elements. You are capable of take quantities of current tuples to create new tuples as the subsequent instance demonstrates −
#!/usr/bin/python
tup1 = (12, 34.56);
tup2 = ('abc', 'xyz');
# Following action is not valid for tuples
# tup1[0] = 100;
# So let's create a new tuple as follows
tup3 = tup1 + tup2;
print tup3;
When the above code is executed, it produces the following result −
(12, 34.56, 'abc', 'xyz')
Delete Tuple Elements
Removing character tuple factors isn’t possible. There is, of course, nothing wrong with placing collectively any other tuple with the undesired factors discarded.
To explicitly eliminate a whole tuple, just use the del announcement. For example −
#!/usr/bin/python
tup = ('physics', 'chemistry', 1997, 2000);
print tup;
del tup;
print "After deleting tup : ";
print tup;
This produces the subsequent end result. Note an exception raised, this is because after del tup tuple does not exist any more −
('physics', 'chemistry', 1997, 2000)
After deleting tup :
Traceback (most recent call last):
File "test.py", line 9, in <module>
print tup;
NameError: name 'tup' is not defined
Python – Tuples, Basic Tuples Operations
Tuples reply to the + and * operators much like strings; they suggest concatenation and repetition here too, except that the end result is a brand new tuple, no longer a string.
In fact, tuples reply to all of the general collection operations we used on strings within the earlier chapter −
Python Expression | Results | Description |
---|---|---|
len((1, 2, 3)) | 3 | Length |
(1, 2, 3) + (4, 5, 6) | (1, 2, 3, 4, 5, 6) | Concatenation |
(‘Hi!’,) * 4 | (‘Hi!’, ‘Hi!’, ‘Hi!’, ‘Hi!’) | Repetition |
3 in (1, 2, 3) | True | Membership |
for x in (1, 2, 3): print x, | 1 2 3 | Iteration |
Python – Tuples, Indexing, Slicing, and Matrixes
Because tuples are sequences, indexing and cutting paintings the same way for tuples as they do for strings. Assuming following input −
L = ('spam', 'Spam', 'SPAM!')
Python Expression | Results | Description |
---|---|---|
L[2] | ‘SPAM!’ | Offsets start at zero |
L[-2] | ‘Spam’ | Negative: count from the right |
L[1:] | [‘Spam’, ‘SPAM!’] | Slicing fetches sections |
Python – Tuples, No Enclosing Delimiters
Python – Tuples, Any set of multiple gadgets, comma-separated, written with out figuring out symbols, i.E., brackets for lists, parentheses for tuples, etc., default to tuples, as indicated in those short examples −
#!/usr/bin/python
print 'abc', -4.24e93, 18+6.6j, 'xyz';
x, y = 1, 2;
print "Value of x , y : ", x,y;
When the above code is accomplished, it produces the subsequent result −
abc -4.24e+93 (18+6.6j) xyz
Value of x , y : 1 2
Built-in Tuple Functions
Python consists of the subsequent tuple features −
Sr.No. | Function with Description |
---|---|
1 | cmp(tuple1, tuple2)Compares elements of both tuples. |
2 | len(tuple)Gives the total length of the tuple. |
3 | max(tuple)Returns item from the tuple with max value. |
4 | min(tuple)Returns item from the tuple with min value. |
5 | tuple(seq)Converts a list into tuple. |