Android AlertDialog, is a small window that activate messages to the user to come to a decision or input additional details. Generally, the Dialogs are used with modals occasion and those beneficial to prompt customers to perform a specific movement to proceed in addition in software.
Following is the pictorial illustration of the use of dialogs in android applications.
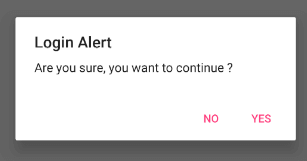
In android, we have a different type of Dialogs available, those are
Dialog | Description |
---|---|
AlertDialog | This dialog is used to display prompt to the user with title, upto three buttons, list of selectable items or a custom layout. |
DatePickerDialog | This dialog is a predefined UI control and it allow user to select Date. |
TimePickerDialog | It’s a predefined UI control and it allow user to select Time. |
In preceding chapters, we protected DatePickerDialog and TimePickerDialog, now we can see how to use AlertDialog in our android applications with examples.
Android AlertDialog
In android, AlertDialog is used to prompt a dialog to the person with message and buttons to perform an action to proceed in addition.
The AlertDialog in android utility will include a 3 regions like as shown below.
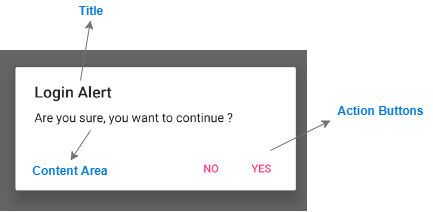
In android Alert Dialogs, we are able to show a name, up to 3 buttons, a list of selectable items or a custom layout based on our necessities.
Region | Description |
---|---|
Title | It’s an optional and it can be used to show the detailed messages based on our requirements. |
Content Area | It is used to display a message, list or other custom layouts based on our requirements. |
Action Buttons | It is used to display an action buttons to interact with the users. We can use upto 3 different action buttons in alert dialog, such as positive, negative and neutral. |
Generally, in android we will build AlertDialog in our pastime record using distinctive conversation strategies.
Android AlertDialog Methods
Following are the some of usually used methods related to AlertDialog manage to constructed alert set off in android programs.
Method | Description |
---|---|
setTitle() | It is used to set the title of alertdialog and its an optional component. |
setIcon() | It is used to set the icon before the title |
setMessage() | It is used to set the message required message to display in alertdialog. |
setCancelable() | It is used to allow users to cancel alertdialog by clicking on outside of dialog area by setting true / false. |
setPositiveButton() | It is used to set the positive button for alertdialog and we can implement click event of positive button. |
setNegativeButton() | It is used to set the negative button for alertdialog and we can implement click event of negative button. |
setNeutralButton() | It is used to set the neutral button for alertdialog and we can implement click event of neutral button. |
Android AlertDialog Example
Following is the instance of defining a one Button control in RelativeLayout to expose the AlertDialog and get the motion which was accomplished by way of consumer on Button click in android software.
Create a new android application the usage of android studio and provide names as AlertDialogExample. In case in case you aren’t aware of creating an app in android studio take a look at this article Android Hello World App.
Now open an activity_main.Xml file from reslayout direction and write the code like as shown under
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<Button
android:id="@+id/getBtn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="150dp"
android:layout_marginTop="200dp"
android:text="Show Alert" />
</RelativeLayout>
If you study above code we defined a one Button manage in RelativeLayout to expose the alert dialog on Button click in XML format document.
Once we’re completed with advent of format with required controls, we want to load the XML format resource from our pastime onCreate() callback approach, for that open predominant pastime file MainActivity.Java from javacom.Tutlane.Alertdialogexample direction and write the code like as proven below.
MainActivity.java
package com.tutlane.alertdialogexample;
import android.content.DialogInterface;
import android.support.v7.app.AlertDialog;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.getBtn);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("Login Alert")
.setMessage("Are you sure, you want to continue ?")
.setCancelable(false)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"Selected Option: YES",Toast.LENGTH_SHORT).show();
}
})
.setNegativeButton("No", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"Selected Option: No",Toast.LENGTH_SHORT).show();
}
});
//Creating dialog box
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
If you examine above code we are calling our layout the use of setContentView technique in the shape of R.Layout.Layout_file_name in our hobby report. Here our xml report call is activity_main.Xml so we used record call activity_main and we try to show the AlertDialog on Button click on.
Generally, throughout the release of our hobby, onCreate() callback method could be referred to as by android framework to get the specified format for an activity.
Output of Android AlertDialog Example
When we run above example the usage of android digital tool (AVD) we can get a end result like as shown beneath.
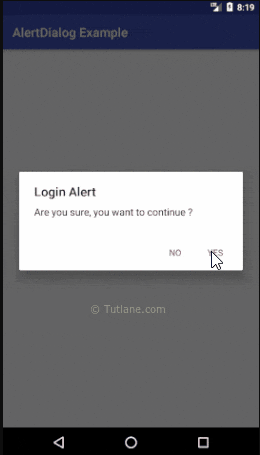
This is how we can use AlertDialog control in android programs to show the alert conversation in android applications based totally on our requirements.
Android AlertDialog Add Items List
In android, we will display the list of items in AlertDialog based totally on our necessities like as proven underneath.
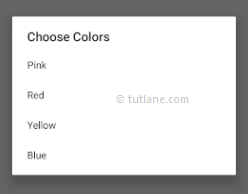
There are 3 different sort of lists available with AlertDialogs in android, those are
Single
- Choice List
- Choice List with Radio Buttons
- Single Choice List with Checkboxes
Now we can see a way to use Single Choice List with Checkboxes in android application to expose the list of objects with checkboxes in AlertDialog and get selected item values with examples.
Android AlertDialog Setmultichoiceitems Example
Create a new android application the use of android studio and supply names as AlertDialogExample. In case if you aren’t aware of developing an app in android studio test this article Android Hello World App.
Now open an activity_main.Xml file from reslayout path and write the code like as shown under
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<Button
android:id="@+id/getBtn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="150dp"
android:layout_marginTop="200dp"
android:text="Show Alert" />
</RelativeLayout>
If you look at above code we defined a one Button manage in RelativeLayout to reveal the alert conversation on Button click in XML format record.
Once we are accomplished with advent of layout with required controls. We want to load the XML layout aid from our pastime onCreate() callback method. For that open important hobby document MainActivity.Java from javacom.Tutlane.Alertdialogexample direction and write the code like as shown beneath.
MainActivity.java
package com.tutlane.alertdialogexample;
import android.content.DialogInterface;
import android.support.v7.app.AlertDialog;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
final CharSequence[] colors = { "Pink", "Red", "Yellow", "Blue" };
ArrayList<Integer> slist = new ArrayList();
boolean icount[] = new boolean[colors.length];
String msg ="";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.getBtn);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setTitle("Choose Colors")
.setMultiChoiceItems(colors,icount, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface arg0, int arg1, boolean arg2) {
if (arg2) {
// If user select a item then add it in selected items
slist.add(arg1);
} else if (slist.contains(arg1)) {
// if the item is already selected then remove it
slist.remove(Integer.valueOf(arg1));
}
}
}) .setCancelable(false)
.setPositiveButton("Yes", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
msg = "";
for (int i = 0; i < slist.size(); i++) {
msg = msg + "\n" + (i + 1) + " : " + colors[slist.get(i)];
}
Toast.makeText(getApplicationContext(), "Total " + slist.size() + " Items Selected.\n" + msg, Toast.LENGTH_SHORT).show();
}
})
.setNegativeButton("No", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"No Option Selected",Toast.LENGTH_SHORT).show();
}
});
//Creating dialog box
AlertDialog dialog = builder.create();
dialog.show();
}
});
}
}
If you observe above code we are calling our layout the use of setContentView approach. Within the shape of R.Layout.Layout_file_name in our pastime file. Here our xml report call is activity_main.Xml so we used document name activity_main. And we try to reveal the list of objects in AlertDialog on Button click on.
Generally, throughout the release of our activity, onCreate() callback method. Can be known as via android framework to get the required layout for an hobby.
Output of Android AlertDialog Example
When we run above instance the usage of android digital tool (AVD) we can get a end result like as shown beneath.
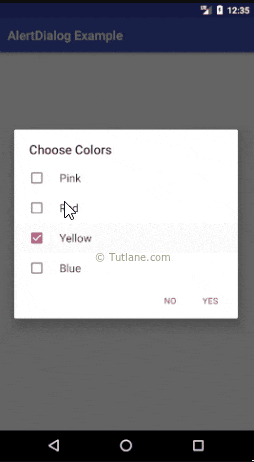
This is how we can use AlertDialog manipulate in android applications to expose the list objects with checkboxes in alert dialog based on our necessities in android programs.