Initial setup
Material Components theme, This is as simple as adding a single Gradle dependency to your app/module build.gradle
file:
implementation “com.google.android.material:material:$material_version“
Material Components for Android is beneath active improvement. The preliminary 1.Zero.Zero launch changed into frequently just a port of the present com.Android.Assist.Layout training over to the brand new com.Google.Android.Cloth namespace.
The first planned independent launch is 1.1.Zero and, on the time of writing, the contemporary model is 1.1.Zero-alpha07. You can music new releases at the GitHub repository.
Choosing a Material Components theme
As with the AppCompat topics, the Material Components issues encompass some base versions as a way to pick out from:
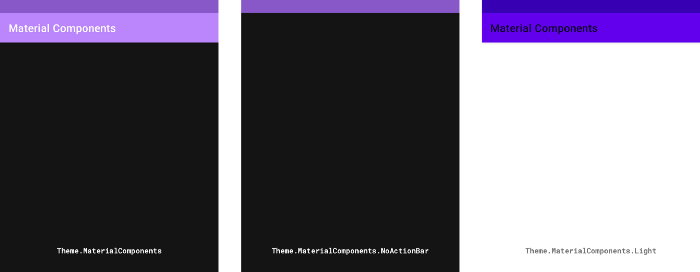
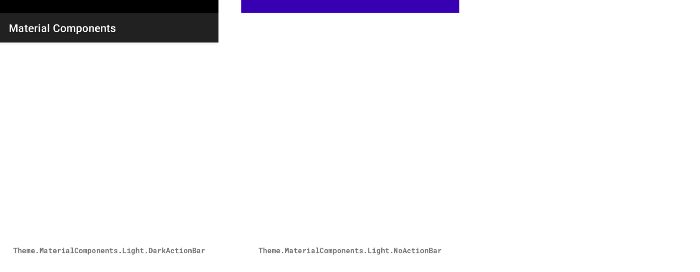
The key variations in every variant are the mild/dark coloration palettes and the inclusion/exclusion of an ActionBar in each themed Activity‘s Window.
Note 1: If you’re migrating an existing subject matter and don’t desire to get all the new attributes straight away, use a Theme.MaterialComponents.*.Bridge variant.
Note 2: Some secondary editions are not shown right here, along with Theme.MaterialComponents.Dialog.* subject matters.
To start the usage of this sort of themes on your app, you’d upload the following on your res/patterns.Xml record:
<style name="AppTheme" parent="Theme.MaterialComponents.*">
<!-- Add attributes here -->
</style>
Finally, you need to reference this in your Manifest:
<manifest
...>
<application
android:theme="@style/AppTheme">
...
</application>
</manifest>
Note: You can also apply an android:theme
per <activity>
in your Manifest.
A simple playground screen
Right, time to get down to enterprise. In order to demonstrate the results of customizing Material Components attributes, we need a visible useful resource.
We may be using the playground display screen underneath, which uses the Theme.MaterialComponents.Light base theme and is full of maximum Material Components widgets and their variations:
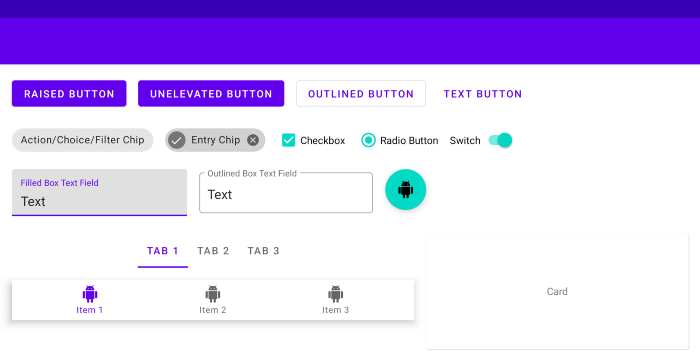
Global theme attributes
The Material Components subject matters introduce new attributes that can be used to fashion elements on a global scale. These can be grouped into three primary subsystems: color, typography and shape.
Color
Color attributes consist specifically of number one, secondary, mistakes, surface and history shades, along with their respective secondary editions and “on” colours. Some of these had been reused from the AppCompat topics (eg. ColorPrimary, colorError and android:colorBackground):
- ColorPrimary: The number one logo shade of your app, used maximum predominantly in theming
- colorPrimaryVariant: A lighter/darker variant of your number one logo colour, used sparingly in theming
- colorOnPrimary: The coloration used for factors displayed on top of your primary colours (eg. Text and icons, often white or semi-obvious black depending on accessibility)
- colorSecondary: The secondary logo colour of your app, used broadly speaking as an accessory for sure widgets that want to face out
- colorSecondaryVariant: A lighter/darker variation of your secondary emblem shade, used sparingly in theming
- colorOnSecondary: The coloration used for factors displayed on top of your secondary shades
- colorError: The colour used for errors (often a coloration of crimson)
- colorOnError: The colour used for elements displayed on top of your blunders coloration
- colorSurface: The colour used for surfaces (i.E. Material “sheets”)
- colorOnSurface: The coloration used for factors displayed on pinnacle of your floor coloration
- android:colorBackground: The coloration behind all other display content material
- colorOnBackground: The colour used for factors displayed on top of your background shade
These colors can be added to your app theme like so:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
<item name="colorPrimary">#212121</item>
<item name="colorPrimaryVariant">#000000</item>
<item name="colorOnPrimary">#FFFFFF</item>
<item name="colorSecondary">#2962FF</item>
<item name="colorSecondaryVariant">#0039CB</item>
<item name="colorOnSecondary">#FFFFFF</item>
<item name="colorError">#F44336</item>
<item name="colorOnError">#FFFFFF</item>
<item name="colorSurface">#FFFFFF</item>
<item name="colorOnSurface">#212121</item>
<item name="android:colorBackground">@color/background</item>
<item name="colorOnBackground">#212121</item>
</style>
<color name="background">#FAFAFA</color>
Note 1: Hex shade codes aren’t presently supported for android:colorBackground, as a result why a shade resource become used.
Note 2: Use android:statusBarColor and android:navigationBarColor attributes to theme gadget bars.
The result may be located in our playground screen:
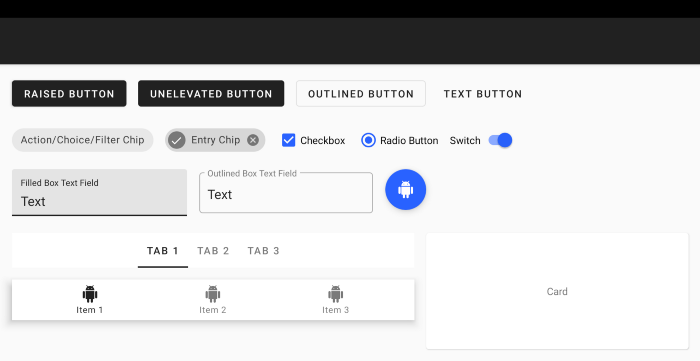
A first rate way to speedy preview the arrival of primary/secondary colorations is to apply the Material Color Tool.
Typography
Type attributes adhere to the Material Type System in phrases of textual content typeface, weight, size, case and letter spacing.
The attributes reference TextAppearance.MaterialComponents.* patterns that put into effect (and are named after) the numerous type scales:
TextAppearance
- Headline
1
: Light, 96sp2
: Light, 60sp3
: Regular, 48sp4
: Regular, 34sp5
: Regular, 24sp6
: Medium, 20spSubtitle1
: Regular, 16spSubtitle2
: Medium, 14spBody1
: Regular, 16spBody2
: Regular, 14spCaption
: Regular, 12spButton
: Regular, 14sp, all capsOverline
: Regular, 12sp, all caps
The Material Components widgets will use those styles as consistent with the Material tips.
You might typically want to keep the default weight, size, case and letter spacing for each fashion. However, a custom typeface can simply make your app stand out.
One might anticipate this requires overriding every and each the sort of attributes. Thankfully, this will be done in a miles greater concise manner via adding the subsequent attributes for your app subject:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="fontFamily">@font/roboto_mono</item>
<item name="android:fontFamily">@font/roboto_mono</item>
</style>
These attributes reference an XML Font or a Downloadable Font which you’ve delivered on your res/font folder and could observe a custom typeface to every widget and text style on your app. There became clearly a time while it wasn’t this clean on Android!
If you do, however, want to customize one of the Material Components text look styles, you will accomplish that like this:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="textAppearanceButton">@style/AppTextAppearance.Button</item>
</style>
<style name="AppTextAppearance.Button" parent="TextAppearance.MaterialComponents.Button">
...
<item name="android:textAllCaps">false</item>
</style>
The results can be observed in our playground screen:
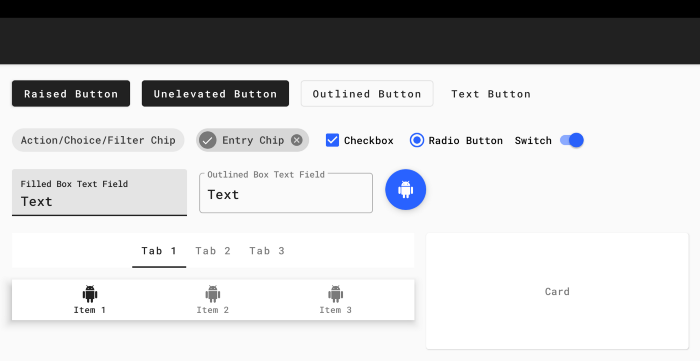
Lastly, Google Fonts is a outstanding location to begin in case you’re searching out unfastened-to-use, custom typefaces (which appear to work certainly properly with Downloadable Fonts too).
Shape
Shape attributes consult with the overall form of each floor and widget on your app. When you don’t forget that these additives can be of varying width/top and be raised/unelevated/outlined, this reduces all the way down to one issue of customization… Corners.
Material Components corners can both be a part of the rounded (default) or cut cornerFamily and have a cornerSize to customise the size. A remedy can be implemented to all corners or a subset. The form topic attributes reference ShapeAppearance.MaterialComponents.* styles:
ShapeAppearance
SmallComponent
: For small components, such as Buttons and ChipsMediumComponent
: Medium components, such as CardsLargeComponent
: For large components, such as Bottom Sheets
The Material Components widgets will use those styles as in step with the Material recommendations.
If you want to customise the Material Components shape look styles, you’ll accomplish that like this:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="shapeAppearanceSmallComponent">@style/AppShapeAppearance.SmallComponent</item>
<item name="shapeAppearanceMediumComponent">@style/AppShapeAppearance.MediumComponent</item>
</style>
<style name="AppShapeAppearance.SmallComponent" parent="ShapeAppearance.MaterialComponents.SmallComponent">
<item name="cornerFamily">cut</item>
<item name="cornerSize">8dp</item>
</style>
<style name="AppShapeAppearance.MediumComponent" parent="ShapeAppearance.MaterialComponents.MediumComponent">
<item name="cornerFamily">cut</item>
<item name="cornerSize">8dp</item>
</style>
The result can be observed in our playground screen:
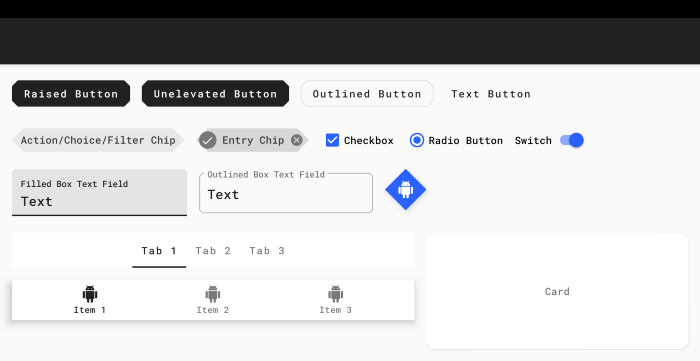
Widget styles and attributes
While global theming covers the majority of our wishes, there are instances while we can also desire to customise the attributes of man or woman widgets.
We will explore the patterns (and applicable attributes) of common widgets and how these can be referenced to your Material Components subject matter.
Buttons
Material Buttons encompass 4 fundamental variations that each one inherit from the bottom Widget.MaterialComponents.Button style.
Each with an non-obligatory fashion suffix: raised (default, no suffix), unelevated (.UnelevatedButton), mentioned (.OutlinedButton) and text (*.TextButton).
All button versions use the textAppearanceButton subject attribute for his or her typography styles.
The key attributes for customizing these styles are as follows:
- BackgroundTint: The tint shade applied to the button history. The default enabled shade is obvious for textual content buttons and colorPrimary for all other variations.
- IconTint: The tint coloration carried out to an elective button icon. The default enabled color is colorPrimary for textual content buttons and colorOnPrimary for all different variations.
- RippleColor: The coloration of the button touch ripple. The default coloration is colorOnPrimary for raised/unelevated buttons and colorPrimary for mentioned/textual content buttons.
- StrokeColor: The shade of the stroke across the button historical past. The default colour is colorOnSurface for mentioned buttons and obvious for all different variants.
- StrokeWidth: The width of the stroke across the button historical past. The default value is 1dp for mentioned buttons and 0dp for all other editions.
- ShapeAppearance: The shape appearance of the button historical past. The default value is shapeAppearanceSmallComponent.
The base button style (used by the MaterialButton
widget class) can be customized and applied globally like so:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="materialButtonStyle">@style/AppButton</item>
</style>
<style name="AppButton" parent="Widget.MaterialComponents.Button">
<item name="backgroundTint">?attr/colorSecondary</item>
</style>
The result can be observed in our playground screen:

Text Fields
Material Text Fields include fundamental variants. As a end result of porting the pre-current AppCompat TextInputLayout and TextInputEditText instructions, there are in reality base styles:
Widget.MaterialComponents.TextInputLayout.* and Widget.MaterialComponents.TextInputEditText.*. The editions have a fashion suffix and include crammed box (default, *.FilledBox) and mentioned field (*.OutlinedBox).
All textual content subject editions use the same old text look for input and the textAppearanceCaption subject attribute for “helper” text (labels, mistakes, counters, and so forth.).
The key attributes for customizing the Widget.MaterialComponents.TextInputLayout.* patterns are as follows:
- boxBackgroundMode: The mode of the field history, which can be either crammed, outline or none.
- BoxBackgroundColor: The coloration of the textual content area heritage. The default enabled coloration is colorOnSurface for filled container text fields and obvious for mentioned field text fields.
- BoxStrokeColor: The shade of the stroke around the textual content field background. The default colour is colorOnSurface (in default nation) for mentioned box text fields and is neglected for stuffed container text fields.
- HintTextColor/errorTextColor/counterTextColor: Various colors for exclusive “helper” text sub-components.
- ShapeAppearance: The form appearance of the text discipline history. The default price is shapeAppearanceSmallComponent.
The base text field style (utilized by the TextInputLayout widget elegance) can be custom designed and applied globally like so:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="textInputStyle">@style/AppTextField</item>
</style>
<style name="AppTextField" parent="Widget.MaterialComponents.TextInputLayout.FilledBox">
<item name="boxBackgroundColor">@color/text_field_background</item>
</style>
Note:text_field_background
is ares/color<selector>
that usescolorSecondary
and the same alpha values as the defaultboxBackgroundColor<selector>
.
The result can be observed in our playground screen:

Material Components theme, Cards
Material Cards are considered to be “surfaces” and employ the Widget.MaterialComponents.CardView fashion. The key attributes for customizing them are as follows:
- cardBackgroundColor: The colour of the card historical past. The default coloration is colorSurface.
- CardElevation: The elevation of the cardboard. The default fee is 1dp.
- ShapeAppearance: The form appearance of the cardboard historical past. The default value is shapeAppearanceMediumComponent.
The base card fashion (used by the MaterialCardView widget magnificence) may be custom designed and implemented globally like so:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="materialCardViewStyle">@style/AppCard</item>
</style>
<style name="AppCard" parent="Widget.MaterialComponents.CardView">
<item name="cardElevation">8dp</item>
</style>
The result can be observed in our playground screen:
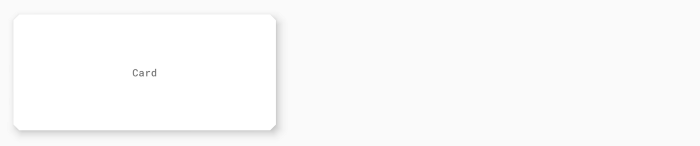
Material Components theme, Bottom Navigation
Material Bottom Navigation includes primary versions that inherit from the base Widget.MaterialComponents.BottomNavigationView style, with an elective fashion suffix: floor (default, no suffix) and coloured (*.Colored).
Bottom Navigation labels use the textAppearanceCaption subject attribute for their typography patterns.
The key attributes for customizing these styles are as follows:
- BackgroundTint: The shade of the lowest navigation heritage. The default color is colorSurface for floor backside navigation and colorPrimary for colored bottom navigation.
- ItemTextColor/itemIconTint: The colours of backside navigation item icons and labels. The default shades are colorOnSurface/colorPrimary(selected) for surface backside navigation and colorOnPrimary for coloured backside navigation.
- ItemHorizontalTranslationEnabled: A flag to set whether or no longer a translation animation need to arise when choosing bottom navigation items. The default price is false.
The base backside navigation style (utilized by the BottomNavigationView widget elegance) may be customized and implemented globally like so:
<style name="AppTheme" parent="Theme.MaterialComponents.Light">
...
<item name="bottomNavigationStyle">@style/AppBottomNavigation</item>
</style>
<style name="AppBottomNavigation" parent="Widget.MaterialComponents.BottomNavigation.Colored" />
The result can be observed in our playground screen:

This is definitely not exhaustive. A extra complete list of all additives and their attributes can be located within the Material Components for Android Docs.
Material Components theme, Build a Material Theme
The Material Components for Android library consists of a module that permits you to easily customise an present Material Theme.
It presents you with a hard and fast of XML files (coloration.Xml/night time/colour.Xml, kind.Xml and form.Xml) which consist of all the necessary baseline subject attributes noted in this article.
The values may be tweaked and previewed in a corresponding sample app. When you’re happy with the selected values, the files can be dropped into a new/current Android Studio project. A web model is also to be had on Glitch.
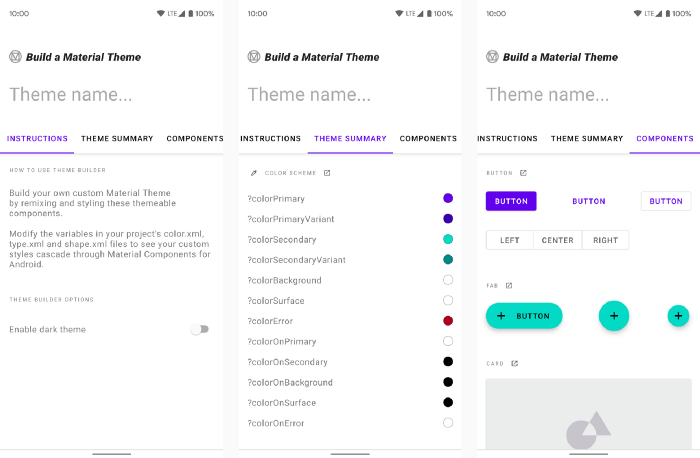