PHP – Web Concepts,This session demonstrates how PHP can offer dynamic content in line with browser type, randomly generated numbers or User Input. It also confirmed how the patron browser may be redirected.
PHP – Web Concepts,Identifying Browser & Platform
PHP creates some beneficial environment variables that can be visible in the phpinfo.Personal home page web page that changed into used to setup the PHP surroundings.
One of the environment variables set by means of PHP is HTTP_USER_AGENT which identifies the user’s browser and running machine.
PHP offers a feature getenv() to access the cost of all of the environment variables. The statistics contained within the HTTP_USER_AGENT surroundings variable may be used to create dynamic content material appropriate to the browser.
Following example demonstrates how you can perceive a consumer browser and operating machine.
NOTE − The function preg_match()is mentioned in PHP Regular expression consultation.
<html>
<body>
<?php
function getBrowser() {
$u_agent = $_SERVER['HTTP_USER_AGENT'];
$bname = 'Unknown';
$platform = 'Unknown';
$version = "";
//First get the platform?
if (preg_match('/linux/i', $u_agent)) {
$platform = 'linux';
}elseif (preg_match('/macintosh|mac os x/i', $u_agent)) {
$platform = 'mac';
}elseif (preg_match('/windows|win32/i', $u_agent)) {
$platform = 'windows';
}
// Next get the name of the useragent yes seperately and for good reason
if(preg_match('/MSIE/i',$u_agent) && !preg_match('/Opera/i',$u_agent)) {
$bname = 'Internet Explorer';
$ub = "MSIE";
} elseif(preg_match('/Firefox/i',$u_agent)) {
$bname = 'Mozilla Firefox';
$ub = "Firefox";
} elseif(preg_match('/Chrome/i',$u_agent)) {
$bname = 'Google Chrome';
$ub = "Chrome";
}elseif(preg_match('/Safari/i',$u_agent)) {
$bname = 'Apple Safari';
$ub = "Safari";
}elseif(preg_match('/Opera/i',$u_agent)) {
$bname = 'Opera';
$ub = "Opera";
}elseif(preg_match('/Netscape/i',$u_agent)) {
$bname = 'Netscape';
$ub = "Netscape";
}
// finally get the correct version number
$known = array('Version', $ub, 'other');
$pattern = '#(?<browser>' . join('|', $known) . ')[/ ]+(?<version>[0-9.|a-zA-Z.]*)#';
if (!preg_match_all($pattern, $u_agent, $matches)) {
// we have no matching number just continue
}
// see how many we have
$i = count($matches['browser']);
if ($i != 1) {
//we will have two since we are not using 'other' argument yet
//see if version is before or after the name
if (strripos($u_agent,"Version") < strripos($u_agent,$ub)){
$version= $matches['version'][0];
}else {
$version= $matches['version'][1];
}
}else {
$version= $matches['version'][0];
}
// check if we have a number
if ($version == null || $version == "") {$version = "?";}
return array(
'userAgent' => $u_agent,
'name' => $bname,
'version' => $version,
'platform' => $platform,
'pattern' => $pattern
);
}
// now try it
$ua = getBrowser();
$yourbrowser = "Your browser: " . $ua['name'] . " " . $ua['version'] .
" on " .$ua['platform'] . " reports: <br >" . $ua['userAgent'];
print_r($yourbrowser);
?>
</body>
</html>
This is generating following end result on my system. This result may be distinctive on your computer relying on what you’re the usage of.
It will produce the following end result −
Your browser: Google Chrome 54.0.2840.99 on windows reports:
Mozilla/5.0 (Windows NT 6.3; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko)
Chrome/54.0.2840.99 Safari/537.36
PHP – Web Concepts,Display Images Randomly
The PHP rand() characteristic is used to generate a random number.I This characteristic can generate numbers with-in a given variety. The random range generator need to be seeded to save you a normal sample of numbers being generated. This is completed using the srand() feature that specifies the seed quantity as its argument.
Following example demonstrates how you can show distinct photograph each time out of 4 snap shots −
<html>
<body>
<?php
srand( microtime() * 1000000 );
$num = rand( 1, 4 );
switch( $num ) {
case 1: $image_file = "/php/images/logo.png";
break;
case 2: $image_file = "/php/images/php.jpg";
break;
case 3: $image_file = "/php/images/logo.png";
break;
case 4: $image_file = "/php/images/php.jpg";
break;
}
echo "Random Image : <img src=$image_file />";
?>
</body>
</html>
It will produce the following result −
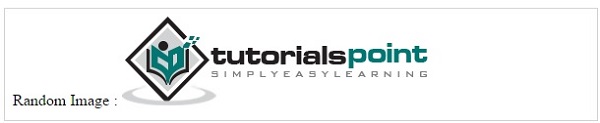
PHP – Web Concepts,Using HTML Forms
The maximum crucial thing to word while handling HTML bureaucracy and PHP is that any form element in an HTML web page will automatically be to be had on your PHP scripts.
Try out following example by way of placing the source code in check.Php script.
<?php
if( $_POST["name"] || $_POST["age"] ) {
if (preg_match("/[^A-Za-z'-]/",$_POST['name'] )) {
die ("invalid name and name should be alpha");
}
echo "Welcome ". $_POST['name']. "<br />";
echo "You are ". $_POST['age']. " years old.";
exit();
}
?>
<html>
<body>
<form action = "<?php $_PHP_SELF ?>" method = "POST">
Name: <input type = "text" name = "name" />
Age: <input type = "text" name = "age" />
<input type = "submit" />
</form>
</body>
</html>
It will produce the following result −

- The PHP default variable $_PHP_SELF is used for the PHP script name and when you click “post” button then equal PHP script may be called and will produce following result −
- The approach = “POST” is used to submit user records to the server script. There are two techniques of posting information to the server script which can be discussed in PHP GET & POST chapter.
Browser Redirection
The PHP header() feature materials raw HTTP headers to the browser and can be used to redirect it to every other region. The redirection script have to be on the very pinnacle of the page to prevent another part of the page from loading.
The target is unique through the Location: header because the argument to the header() function. After calling this characteristic the exit() feature may be used to halt parsing of relaxation of the code.
Following instance demonstrates how you can redirect a browser request to every other web page. Try out this case through setting the supply code in test.Php script.
<?php
if( $_POST["location"] ) {
$location = $_POST["location"];
header( "Location:$location" );
exit();
}
?>
<html>
<body>
<p>Choose a site to visit :</p>
<form action = "<?php $_SERVER['PHP_SELF'] ?>" method ="POST">
<select name = "location">.
<option value = "http://www.tutorialspoint.com">
Tutorialspoint.com
</option>
<option value = "http://www.google.com">
Google Search Page
</option>
</select>
<input type = "submit" />
</form>
</body>
</html>
It will produce the following result −
