PHP Error Handling Guide, Because PHP is free to apply, many web developers use this programming language to make web applications. Even the maximum popular internet content material management systems are primarily based on this programming language.
Handling mistakes in PHP is almost the same as handling mistakes in other programming languages. But if a developer is new to the language, seeing the errors for the first time may be scary and annoying. Below is an instance.
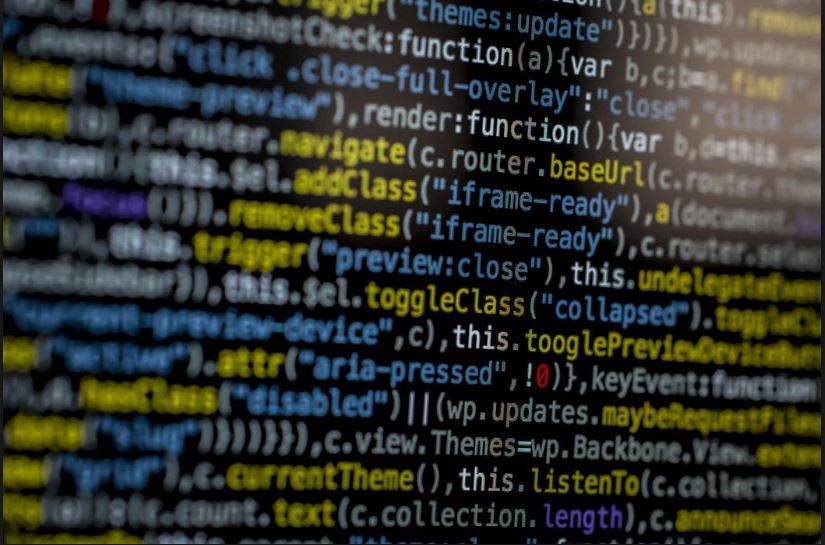
if $x==1 {
echo "Hello World!";
}
Parse error: syntax error, unexpected '$x' (T_VARIABLE), expecting '('
PHP Error Handling Guide, Syntax blunders messages in PHP are comparable to syntax mistakes in other languages. Anyone with the knowledge about this language can be capable of hint and attach this error.
PHP Error Handling Tutorial
PHP Error Handling Guide,In this text, you’ll learn about the distinct forms of errors including blunders logs, errors coping with, error handling capabilities, and different blunders PHP framework blunders coping with. Let’s begin via defining the distinction between the phrases “error” and “exception” and other commonplace terms in PHP.
Errors vs. Exceptions
PHP Error Handling Guide, Many use error managing and exception dealing with interchangeably. When we are saying mistakes coping with we’re regarding the method of catching errors produced by means of your program which wishes proper motion.
Since PHP came into a brand new object-oriented (OOP) manner of managing mistakes, exception managing become delivered. It is used to alternate the usual manner of coping with code execution of a particular blunders situation whilst it happens. In this manner, exception coping with provides a higher technique over errors coping with.
How does exception managing in PHP work?
Just like every other object-orientated programming, PHP also makes use of the subsequent key phrases associated with exceptions:
Try: which means that if the exception does not cause, the code will simply execute commonly however if the exception triggers then it’ll name “thrown” exception
Throw: every time an exception has been precipitated, a “throw” exception should be paired with at the least one “catch”
Catch: this block of code should retrieve an exception and create an item including the exception information.
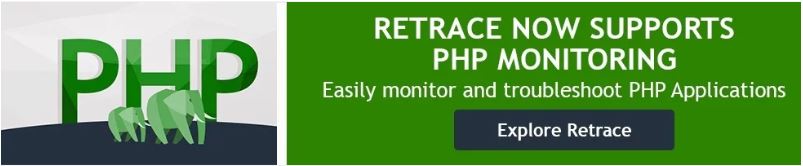
Error logs
PHP Error Handling Guide, Error logs are crucial at some stage in development because this lets in builders to look the errors, caution, notices, and so forth. That were logged even as the application is strolling.
Most of the time, when an internet application is deployed, all mistakes and warnings are suppressed to prevent ordinary customers from seeing the mistakes that will arise.
Even even though the errors and warnings are hidden from the ordinary users, the internet server still logs each event the website encounters.
Simple error handling
Whenever the utility is in an wrong state wherein it can not recover, then this system is in an errors country.
// Set MySQLi to throw exceptions
mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT);
try {
$connection2 = mysqli_connect('localhost', 'root1', 'Abc123456!', 'testx');
} catch (mysqli_sql_exception $ex) {
throw new Exception("Can't connect to the database! \n" . $ex);
}
Fatal error: Uncaught Exception: Can't connect to the database! mysqli_sql_exception: Access denied for user 'root1'@'localhost' to database 'testx'
Because the parameters for the mysqli_connect are incorrect, the quality way to deal with this form of state of affairs is the program throwing an exception.
It’s useless that the application hold executing because it’d no longer still go back a few statistics from the database.
Using die() or exit() for handling errors
mysqli_report(MYSQLI_REPORT_ERROR | MYSQLI_REPORT_STRICT);
try {
$connection2 = mysqli_connect('localhost', 'root1', 'Abc123456!', 'testx');
} catch (mysqli_sql_exception $ex) {
die("Can't connect to the database! \n" . $ex);
}
During improvement, using the feature die or go out to halt code is a move-to-coding fashion for builders. In code and even tutorials, whilst connecting through a database, if the relationship is inaccurate or invalid, then the application calls both of those two features to halt the execution of the program.
This could be very beneficial in managing mistakes and tracing code, but when the utility is deployed, this might scare the ordinary customers of the internet utility.
Errors like “Fatal blunders: Uncaught Exception: Can’t connect with the database! Mysqli_sql_exception” isn’t always beneficial to the end consumer.
When the code that handles the database connection makes use of die or go out, then there is no manner to suppress these errors messages at the stay net server. Exceptions, notices, and many others.
Can be hidden with only a simple net server configuration or additional PHP code that’s why throwing exceptions is greater practical in managing PHP errors.
Retrace error logging
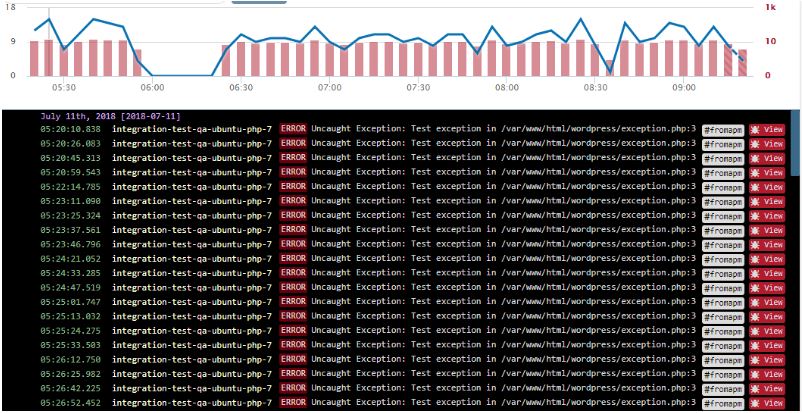
In Retrace, all mistakes are recorded together with some important statistics about that mistakes like the time it passed off, the technique that induced it, and the exception it generated. The quantity of instances the error came about in a particular time body is also graphed.
From this records, builders can easily take a look at what brought about the mistake and then practice a repair. Project managers can also prioritize which trouble to repair based at the quantity of occurrence of an blunders.
PHP error handling functions
function myTestFunctionHello($str)f
{
echo "\nHi: $str";
myTestFunctionGoodbye($str);
}
function myTestFunctionGoodbye($str)
{
echo "\nGoodbye: $str";
print_r(debug_backtrace());
}
myTestFunctionHello('World');
// output
{[0]=> array(4) { ["file"]=> string(43) "\var\www\test-error.php" ["line"]=> int(6) ["function"]=> string(21) "myTestFunctionGoodbye" ["args"]=> array(1)
{ [0]=> string(5) "World" }
}
[1]=> array(4){ ["file"]=> string(43) "\var\www\test-error.php" ["line"]=> int(15) ["function"]=> string(19) "myTestFunctionHello" ["args"]=> array(1)
{ [0]=> string(5) "World" }
}}
The debug_backtrace lets in builders to look which feature changed into known as first. Essentially, this can go back an array of characteristic calls, lines, objects, class, and many others.
This is helpful in tracing errors because any restoration made can affect the opposite functions that calls or use it. A similar feature, debug_print_backtrace, also offers similar output however with more details.
echo $unknown_a;
echo $unknown_b;
print_r(error_get_last());
Array ( [type] => 8 [message] => Undefined variable: unknown_b [file] => C:\xampp\htdocs\monolog-test\test-error.php
=> 4 )
The function error_get_last will get the remaining errors within the stack hint. This can be used to take away all the different errors displayed in the stack trace and most effective focus at the final error that has befell.
// will log to the web server error log file, will also log to Retrace
error_log("This is a sample error.", 0);
// will email the error log
error_log("This is a sample error.", 1, "[email protected]");
// will log an error to a specified file
error_log("This is a sample error.", 3, "my-errors.log");
The error_log characteristic allows builders to log errors in both the default error log of the web server, send the error log to an e-mail, or log the mistake to a distinct file.
$divisor = 0;
if ($divisor == 0) {
trigger_error("Division of zero not allowed!", E_USER_ERROR);
}
Fatal error: Division of zero not allowed! in C:\xampp\htdocs\monolog-test\test-error.php on line 5
This will generate a user described errors, word, warning etc. This PHP function allows developers to generate a special reaction at runtime.
Other PHP framework error handling
In different PHP frameworks, blunders coping with is a lot less difficult and maximum of them are just a matter of modifying the configuration document.
In Laravel, the class AppExceptionsHandler handles all the caused exceptions and logging by means of an internet application. The Handler elegance in Laravel has two techniques, file and render.
The file approach is used to ship exceptions to an outside service. The record is right for logging mistakes without displaying it in a web web page. The render method will convert an exception into an HTTP response that needs to be sent again to the browser.
abort(500);
abort(403, 'You are not allowed to enter!');
Throwing HTTP exceptions can without difficulty be executed in Laravel by way of using the abort helper. The abort helper characteristic has an non-compulsory reaction text parameter for a custom display message.
Conclusion
Errors and troubleshooting take time and headache during development and even within the deployment of your app. The proper device to deal with errors makes your life less difficult. Stackify combines debug item logging with errors logging, which could without difficulty take a look at the basis reason of your exceptions. Stackify’s errors and log control tool permit you to without problems screen and troubleshoot your software. You can try our 14 day unfastened trial now!