Android Toast, Toast is a small popup notification. Which is used to display an information approximately the operation which we performed in our app. The Toast will show the message for a small period of time and it’ll disappear routinely after a timeout.
Generally, the size of Toast could be adjusted based totally on the distance. Required for the message and it will be displayed. On the top of essential content material of an interest for a quick time frame.
For example, some of the apps will show a message like “Press again to go out” in toast. While we pressed a back button within the home page or showing a message like “stored effectively” toast. Whilst we click on button to store the details.
Following is the pictorial illustration of using Toast in android programs.In android. Toast is a small popup notification which is used to show. An facts approximately the operation which we accomplished in our app. The Toast will show the message for a small time frame and it will disappear automatically after a timeout.
Generally, the dimensions of Toast will be adjusted primarily based. On the distance required for the message and it is going to be displayed. At the top of fundamental content material of an activity for a quick time period.
For example, some of the apps will show a message like “Press once more to exit” in toast. When we pressed a again button in the home web page or showing a message like “saved efficaciously” toast. Whilst we click on button to shop the info.
Following is the pictorial representation of using Toast in android programs.
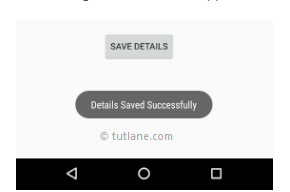
Create a Toast in Android
In android, we are able to create a Toast by way of instantiating an android. Widget. Toast object the usage of makeText() approach. The makeText() technique will take 3 parameters: software context, text message and the length for the toast. We can show the Toast notification by means of using display() technique.
Following is the syntax of making a Toast in android packages.
Toast.makeText(context, "message", duration).show();
If you look at above syntax, we defined a Toast notification. The use of makeText() method with 3 parameters, those are
Parameter | Description |
---|---|
context | It’s our application context. |
message | It’s our custom message which we want to show in Toast notification. |
duration | It is used to define the duration for notification to display on screen. |
We have a approaches to define the Toast length. Both in LENGTH_SHORT or LENGTH_LONG to display the toast notification for short or longer time frame.
Following is the instance of defining a Toast in android applications.
Toast.makeText(MainActivity.this, "Details Saved Successfully.", Toast.LENGTH_SHORT).show();
Now we will see how to implement a Toast notification in android applications with examples.
Android Toast Notification Example
Create a new android application using android studio and provide names as ToastExample. In case in case you aren’t privy to growing an app in android studio. Test this text Android Hello World App.
Now open an activity_main.Xml record from reslayout path and write the code like as shown under
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<Button
android:id="@+id/btnShow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Toast"
android:layout_marginTop="200dp" android:layout_marginLeft="140dp"/>
</LinearLayout>
If you take a look at above code we created a one Button control in XML Layout documen. To reveal the toast notification when we click on Button.
Once we are achieved with advent of format with required controls. We need to load the XML format useful resource from our activity onCreate() callback approach. For that open primary pastime file MainActivity.Java from javacom.Tutlane.Toastexample route and write the code like as proven beneath.
MainActivity.java
package com.tutlane.toastexample;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.btnShow);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this, "You Clicked on Button..", Toast.LENGTH_SHORT).show();
}
});
}
}
If you study above code we’re created a toast notification. The use of makeText() method and showing a toast notification on Button click on.
Generally, throughout the launch of our activity, onCreate() callback. Approach may be called with the aid of android framework to get the specified layout for an hobby.
Output of Android Toast Notification Example
When we run above example using android virtual device (AVD) we will get a result like as shown below.
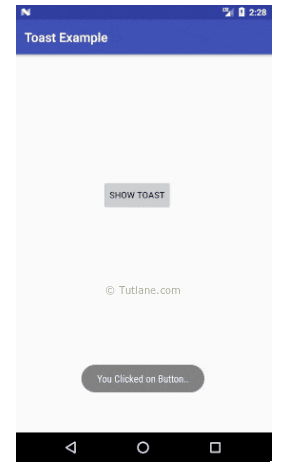
If you observe above result we created a toast notification and shown it on Button click based on our requirements.
Change the Position of Android Toast Notification
By default, the android Toast notification will continually seem near the bottom of the display. Targeted horizontally like as proven in above picture.
In case if we want to change the position of Toast notification. We are able to do it by way of the usage of setGravity(int, int, int) approach. The setGravity() technique will accepts three parameters: a Gravity constant, an x-role offset, and a y-position offset.
Following is the instance of converting the location of android Toast notification. To top-right based on offset positions via the use of setGravity() approach.
Toast toast = Toast.makeText(MainActivity.this, "You Clicked on Button..", Toast.LENGTH_SHORT);
toast.setGravity(Gravity.TOP|Gravity.RIGHT, 100, 250);
toast.show();
If you need to move the placement of toast to proper, growth the value of the second parameter. To flow it down, increase the cost of the closing parameter.
Android Toast Positioning Example
Following is the example of converting the position of android toast notification to top-proper facet the use of setGravity() technique.
We want to regulate our principal interest record MainActivity.Java code like as shown under.
MainActivity.java
package com.tutlane.toastexample;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Gravity;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.btnShow);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//display toast message in top right side
Toast toast = Toast.makeText(MainActivity.this, "You Clicked on Button..", Toast.LENGTH_SHORT);
toast.setGravity(Gravity.TOP|Gravity.RIGHT, 100, 250);
toast.show();
}
});
}
}
If you observe above code we are changing the position of android toast notification using setGravity() property.
Output of Android Toast Positioning Example
When we run above example using android virtual device (AVD) we will get a result like as shown below.
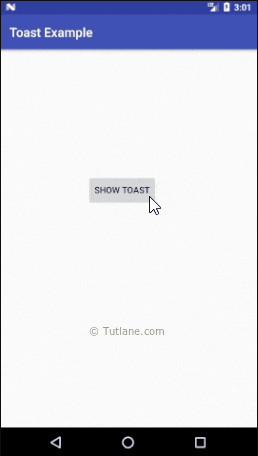
If you look at above result we changed the location of toast notification. To top right aspect on Button click primarily based on our necessities.
In case if we want to alternate the fashion of toast notification. Then we can do it by using creating a custom XML layout file. To recognize more about custom toast view, test this Android Custom Toast with Example.